UNIVERSITY OF NORTHERN BRITISH
COLUMBIA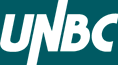
Winter 2002 Computer Science
Code
Generator Examples
| |
Program 1:
euclid.mns
/***
A program to perform Euclid's Algorithm to compute gcd.
***/
int gcd (int u, int v)
{
if (v == 0)
return u ;
else
return gcd(v,u-u/v*v);
/* u-u/v*v == u mod v */
}
void main(void)
{ int x; int y;
x = input(); y = input();
output(gcd(x,y));
} Object Code
Output #1
* TINY Compilation to TM Code
* File: euclid.tm
* Standard prelude:
0: LD 6,0(0) load maxaddress from location 0 into gp
1: LDA 5,0(6) copy gp to fp
2: ST 0,0(0) clear location 0
* End of standard prelude.
* gcd
7: ST 0,-1(5) store the return pointer
* -> if
* -> Op
* -> Id
8: LD 0,2(5) Load value into the ac
* <- Id
9: ST 0,-3(5) op: push left
* -> Const
10: LDC 0,0(0) load const
* <- Const
11: LD 1,-3(5) op: load left
12: SUB 0,1,0 op ==
13: JEQ 0,2(7) br if true
14: LDC 0,0(0) false case
15: LDA 7,1(7) unconditional jmp
16: LDC 0,1(0) true case
* <- Op
* if: jump to else belongs here
* -> Id
18: LD 0,-2(5) Load value into the ac
* <- Id
19: LD 7,-1(5) load return pointer into pc
* if: jump to end belongs here
17: JEQ 0,3(7) if: jmp to else
* gcd
* -> Id
21: LD 0,2(5) Load value into the ac
* <- Id
22: ST 0,-5(5) adding arg to activation record
* -> Op
* -> Id
23: LD 0,-2(5) Load value into the ac
* <- Id
24: ST 0,-6(5) op: push left
* -> Op
* -> Op
* -> Id
25: LD 0,-2(5) Load value into the ac
* <- Id
26: ST 0,-7(5) op: push left
* -> Id
27: LD 0,2(5) Load value into the ac
* <- Id
28: LD 1,-7(5) op: load left
29: DIV 0,1,0 op /
* <- Op
30: ST 0,-7(5) op: push left
* -> Id
31: LD 0,2(5) Load value into the ac
* <- Id
32: LD 1,-7(5) op: load left
33: MUL 0,1,0 op *
* <- Op
34: LD 1,-6(5) op: load left
35: SUB 0,1,0 op -
* <- Op
36: ST 0,-6(5) adding arg to activation record
37: ST 5,-3(5) store current fp
38: LDA 5,-3(5) push new frame
39: LDA 0,1(7) save return in ac
40: LDA 7,-34(7) Relative jump to current func
41: LD 5,0(5) pop current frame
* gcd
42: LD 7,-1(5) load return pointer into pc
20: LDA 7,22(7) jmp to end
* <- if
43: LD 7,-1(5) load return pointer into pc
* gcd
* main
44: ST 0,-1(5) store the return pointer
* -> assign
* -> Id
45: LDA 0,-2(5) Load address into the ac
* <- Id
46: ST 0,-4(5) =: push left address
47: IN 0,0,0 input integer value
48: LD 1,-4(5) op: load left
49: ST 0,0(1) assign: store value
* <- assign
* -> assign
* -> Id
50: LDA 0,-3(5) Load address into the ac
* <- Id
51: ST 0,-4(5) =: push left address
52: IN 0,0,0 input integer value
53: LD 1,-4(5) op: load left
54: ST 0,0(1) assign: store value
* <- assign
* gcd
* -> Id
55: LD 0,-2(5) Load value into the ac
* <- Id
56: ST 0,-6(5) adding arg to activation record
* -> Id
57: LD 0,-3(5) Load value into the ac
* <- Id
58: ST 0,-7(5) adding arg to activation record
59: ST 5,-4(5) store current fp
60: LDA 5,-4(5) push new frame
61: LDA 0,1(7) save return in ac
62: LDA 7,-56(7) Relative jump to current func
63: LD 5,0(5) pop current frame
* gcd
64: OUT 0,0,0 output ac
65: LD 7,-1(5) load return pointer into pc
* main
* Calling sequence for main
3: LDA 5,0(5) push frame main
4: LDA 0,1(7) save return in ac for main
5: LDA 7,38(7) Relative jump to main
* End of execution.
6: HALT 0,0,0 Correct Halt
Program 2: nfact.mns
/**************
recursive N factorial. Used
for testing recursion.
**************/
/* recursively call n!*/
int nfact(int n)
{
if( n == 0 )
{
return 1;
}
else
{
return(n*nfact(n-1));
}
return 0;
}
void main(void)
{
int n;
n = input();
n = nfact(n);
output(n);
}
Object Code Output #2
* TINY Compilation to TM Code
* File: nfact.tm
* Standard prelude:
0: LD 6,0(0) load maxaddress from location 0 into gp
1: LDA 5,0(6) copy gp to fp
2: ST 0,0(0) clear location 0
* End of standard prelude.
* nfact
7: ST 0,-1(5) store the return pointer
* -> if
* -> Op
* -> Id
8: LD 0,-2(5) Load value into the ac
* <- Id
9: ST 0,-3(5) op: push left
* -> Const
10: LDC 0,0(0) load const
* <- Const
11: LD 1,-3(5) op: load left
12: SUB 0,1,0 op ==
13: JEQ 0,2(7) br if true
14: LDC 0,0(0) false case
15: LDA 7,1(7) unconditional jmp
16: LDC 0,1(0) true case
* <- Op
* if: jump to else belongs here
* -> Const
18: LDC 0,1(0) load const
* <- Const
19: LD 7,-1(5) load return pointer into pc
* if: jump to end belongs here
17: JEQ 0,3(7) if: jmp to else
* -> Op
* -> Id
21: LD 0,-2(5) Load value into the ac
* <- Id
22: ST 0,-3(5) op: push left
* nfact
* -> Op
* -> Id
23: LD 0,-2(5) Load value into the ac
* <- Id
24: ST 0,-6(5) op: push left
* -> Const
25: LDC 0,1(0) load const
* <- Const
26: LD 1,-6(5) op: load left
27: SUB 0,1,0 op -
* <- Op
28: ST 0,-6(5) adding arg to activation record
29: ST 5,-4(5) store current fp
30: LDA 5,-4(5) push new frame
31: LDA 0,1(7) save return in ac
32: LDA 7,-26(7) Relative jump to current func
33: LD 5,0(5) pop current frame
* nfact
34: LD 1,-3(5) op: load left
35: MUL 0,1,0 op *
* <- Op
36: LD 7,-1(5) load return pointer into pc
20: LDA 7,16(7) jmp to end
* <- if
* -> Const
37: LDC 0,0(0) load const
* <- Const
38: LD 7,-1(5) load return pointer into pc
39: LD 7,-1(5) load return pointer into pc
* nfact
* main
40: ST 0,-1(5) store the return pointer
* -> assign
* -> Id
41: LDA 0,-2(5) Load address into the ac
* <- Id
42: ST 0,-3(5) =: push left address
43: IN 0,0,0 input integer value
44: LD 1,-3(5) op: load left
45: ST 0,0(1) assign: store value
* <- assign
* -> assign
* -> Id
46: LDA 0,-2(5) Load address into the ac
* <- Id
47: ST 0,-3(5) =: push left address
* nfact
* -> Id
48: LD 0,-2(5) Load value into the ac
* <- Id
49: ST 0,-6(5) adding arg to activation record
50: ST 5,-4(5) store current fp
51: LDA 5,-4(5) push new frame
52: LDA 0,1(7) save return in ac
53: LDA 7,-47(7) Relative jump to current func
54: LD 5,0(5) pop current frame
* nfact
55: LD 1,-3(5) op: load left
56: ST 0,0(1) assign: store value
* <- assign
* -> Id
57: LD 0,-2(5) Load value into the ac
* <- Id
58: OUT 0,0,0 output ac
59: LD 7,-1(5) load return pointer into pc
* main
* Calling sequence for main
3: LDA 5,0(5) push frame main
4: LDA 0,1(7) save return in ac for main
5: LDA 7,34(7) Relative jump to main
* End of execution.
6: HALT 0,0,0 Correct Halt
Program 3: quicksort.mns
Quicksort
alogirthm routine
*/
void swap( int arr[], int i, int j )
{
int temp;
temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
return;
}
int partition( int arr[], int lo, int hi )
{ /* hi is the highest index to use, lo is the lowest index to use */
int sz;
int mid;
int median;
int temp;
sz = hi - lo;
mid = lo + (hi - lo)/2;
/* median of three partitioning */
if( arr[lo] > arr[mid] )
{
swap( arr, lo, mid );
}
if( arr[lo] > arr[hi] )
{
swap( arr, lo, hi );
}
if( arr[mid] > arr[hi] )
{
swap( arr, mid, hi );
}
swap(arr,mid, hi-1);
return ( arr[hi-1] );
}
void quicksort( int arr[], int lo, int hi )
{
int part;
int lb;
int hb;
if(lo + 2 < hi)
{
int pivot;
int flag;
int i;
int j;
flag = 0;
pivot = partition(arr, lo, hi );
i = lo;
j = hi - 1;
while( flag == 0 )
{
i = i + 1;
while ( arr[i] < pivot )
{
i = i + 1;
}
j = j - 1;
while( arr[j] > pivot )
{
j = j - 1;
}
if ( i < j )
{
swap( arr, i, j );
}
else
{
flag = 1;
}
}
swap( arr, i, hi - 1 );
quicksort(arr, lo, i-1 );
quicksort(arr, i+1, hi );
}
else
{
/* replace insertion sort*/
int mid;
mid = (lo + hi) /2;
if( arr[lo] > arr[mid] )
{
swap( arr, lo, mid );
}
if( arr[lo] > arr[hi] )
{
swap( arr, lo, hi );
}
if( arr[mid] > arr[hi] )
{
swap( arr, mid, hi );
}
}
return;
} Object Code Output
#3
* TINY Compilation to TM Code
* File: quicksort.tm
* Standard prelude:
0: LD 6,0(0) load maxaddress from location 0 into gp
1: LDA 5,0(6) copy gp to fp
2: ST 0,0(0) clear location 0
* End of standard prelude.
* swap
7: ST 0,-1(5) store the return pointer
* -> assign
* -> Id
8: LDA 0,-5(5) Load address into the ac
* <- Id
9: ST 0,-6(5) =: push left address
* -> Id
* -> Id
10: LD 0,-3(5) Load value into the ac
* <- Id
11: LD 1,-2(5) Load array param address into ac1
12: SUB 0,1,0 Add index to base array address
13: LD 0,0(0) Loading value of array index into ac
* <- Id
14: LD 1,-6(5) op: load left
15: ST 0,0(1) assign: store value
* <- assign
* -> assign
* -> Id
* -> Id
16: LD 0,-3(5) Load value into the ac
* <- Id
17: JGE 0,1(7) if array index < 0, halt
18: HALT 0,0,0 Negative array index
19: LD 1,-2(5) Load array param address into ac1
20: SUB 0,1,0 Add index to base array address
* <- Id
21: ST 0,-6(5) =: push left address
* -> Id
* -> Id
22: LD 0,-4(5) Load value into the ac
* <- Id
23: LD 1,-2(5) Load array param address into ac1
24: SUB 0,1,0 Add index to base array address
25: LD 0,0(0) Loading value of array index into ac
* <- Id
26: LD 1,-6(5) op: load left
27: ST 0,0(1) assign: store value
* <- assign
* -> assign
* -> Id
* -> Id
28: LD 0,-4(5) Load value into the ac
* <- Id
29: JGE 0,1(7) if array index < 0, halt
30: HALT 0,0,0 Negative array index
31: LD 1,-2(5) Load array param address into ac1
32: SUB 0,1,0 Add index to base array address
* <- Id
33: ST 0,-6(5) =: push left address
* -> Id
34: LD 0,-5(5) Load value into the ac
* <- Id
35: LD 1,-6(5) op: load left
36: ST 0,0(1) assign: store value
* <- assign
37: LD 7,-1(5) load return pointer into pc
38: LD 7,-1(5) load return pointer into pc
* swap
* partition
39: ST 0,-1(5) store the return pointer
* -> assign
* -> Id
40: LDA 0,-5(5) Load address into the ac
* <- Id
41: ST 0,-9(5) =: push left address
* -> Op
* -> Id
42: LD 0,-4(5) Load value into the ac
* <- Id
43: ST 0,-10(5) op: push left
* -> Id
44: LD 0,-3(5) Load value into the ac
* <- Id
45: LD 1,-10(5) op: load left
46: SUB 0,1,0 op -
* <- Op
47: LD 1,-9(5) op: load left
48: ST 0,0(1) assign: store value
* <- assign
* -> assign
* -> Id
49: LDA 0,-6(5) Load address into the ac
* <- Id
50: ST 0,-9(5) =: push left address
* -> Op
* -> Id
51: LD 0,-3(5) Load value into the ac
* <- Id
52: ST 0,-10(5) op: push left
* -> Op
* -> Op
* -> Id
53: LD 0,-4(5) Load value into the ac
* <- Id
54: ST 0,-11(5) op: push left
* -> Id
55: LD 0,-3(5) Load value into the ac
* <- Id
56: LD 1,-11(5) op: load left
57: SUB 0,1,0 op -
* <- Op
58: ST 0,-11(5) op: push left
* -> Const
59: LDC 0,2(0) load const
* <- Const
60: LD 1,-11(5) op: load left
61: DIV 0,1,0 op /
* <- Op
62: LD 1,-10(5) op: load left
63: ADD 0,1,0 op +
* <- Op
64: LD 1,-9(5) op: load left
65: ST 0,0(1) assign: store value
* <- assign
* -> if
* -> Op
* -> Id
* -> Id
66: LD 0,-3(5) Load value into the ac
* <- Id
67: LD 1,-2(5) Load array param address into ac1
68: SUB 0,1,0 Add index to base array address
69: LD 0,0(0) Loading value of array index into ac
* <- Id
70: ST 0,-9(5) op: push left
* -> Id
* -> Id
71: LD 0,-6(5) Load value into the ac
* <- Id
72: LD 1,-2(5) Load array param address into ac1
73: SUB 0,1,0 Add index to base array address
74: LD 0,0(0) Loading value of array index into ac
* <- Id
75: LD 1,-9(5) op: load left
76: SUB 0,1,0 op >
77: JGT 0,2(7) br if true
78: LDC 0,0(0) false case
79: LDA 7,1(7) unconditional jmp
80: LDC 0,1(0) true case
* <- Op
* if: jump to else belongs here
* swap
* -> Id
82: LD 0,-2(5) Load array param address into ac
* <- Id
83: ST 0,-11(5) adding arg to activation record
* -> Id
84: LD 0,-3(5) Load value into the ac
* <- Id
85: ST 0,-12(5) adding arg to activation record
* -> Id
86: LD 0,-6(5) Load value into the ac
* <- Id
87: ST 0,-13(5) adding arg to activation record
88: ST 5,-9(5) store current fp
89: LDA 5,-9(5) push new frame
90: LDA 0,1(7) save return in ac
91: LDA 7,-85(7) Relative jump to current func
92: LD 5,0(5) pop current frame
* swap
* if: jump to end belongs here
81: JEQ 0,12(7) if: jmp to else
93: LDA 7,0(7) jmp to end
* <- if
* -> if
* -> Op
* -> Id
* -> Id
94: LD 0,-3(5) Load value into the ac
* <- Id
95: LD 1,-2(5) Load array param address into ac1
96: SUB 0,1,0 Add index to base array address
97: LD 0,0(0) Loading value of array index into ac
* <- Id
98: ST 0,-9(5) op: push left
* -> Id
* -> Id
99: LD 0,-4(5) Load value into the ac
* <- Id
100: LD 1,-2(5) Load array param address into ac1
101: SUB 0,1,0 Add index to base array address
102: LD 0,0(0) Loading value of array index into ac
* <- Id
103: LD 1,-9(5) op: load left
104: SUB 0,1,0 op >
105: JGT 0,2(7) br if true
106: LDC 0,0(0) false case
107: LDA 7,1(7) unconditional jmp
108: LDC 0,1(0) true case
* <- Op
* if: jump to else belongs here
* swap
* -> Id
110: LD 0,-2(5) Load array param address into ac
* <- Id
111: ST 0,-11(5) adding arg to activation record
* -> Id
112: LD 0,-3(5) Load value into the ac
* <- Id
113: ST 0,-12(5) adding arg to activation record
* -> Id
114: LD 0,-4(5) Load value into the ac
* <- Id
115: ST 0,-13(5) adding arg to activation record
116: ST 5,-9(5) store current fp
117: LDA 5,-9(5) push new frame
118: LDA 0,1(7) save return in ac
119: LDA 7,-113(7) Relative jump to current func
120: LD 5,0(5) pop current frame
* swap
* if: jump to end belongs here
109: JEQ 0,12(7) if: jmp to else
121: LDA 7,0(7) jmp to end
* <- if
* -> if
* -> Op
* -> Id
* -> Id
122: LD 0,-6(5) Load value into the ac
* <- Id
123: LD 1,-2(5) Load array param address into ac1
124: SUB 0,1,0 Add index to base array address
125: LD 0,0(0) Loading value of array index into ac
* <- Id
126: ST 0,-9(5) op: push left
* -> Id
* -> Id
127: LD 0,-4(5) Load value into the ac
* <- Id
128: LD 1,-2(5) Load array param address into ac1
129: SUB 0,1,0 Add index to base array address
130: LD 0,0(0) Loading value of array index into ac
* <- Id
131: LD 1,-9(5) op: load left
132: SUB 0,1,0 op >
133: JGT 0,2(7) br if true
134: LDC 0,0(0) false case
135: LDA 7,1(7) unconditional jmp
136: LDC 0,1(0) true case
* <- Op
* if: jump to else belongs here
* swap
* -> Id
138: LD 0,-2(5) Load array param address into ac
* <- Id
139: ST 0,-11(5) adding arg to activation record
* -> Id
140: LD 0,-6(5) Load value into the ac
* <- Id
141: ST 0,-12(5) adding arg to activation record
* -> Id
142: LD 0,-4(5) Load value into the ac
* <- Id
143: ST 0,-13(5) adding arg to activation record
144: ST 5,-9(5) store current fp
145: LDA 5,-9(5) push new frame
146: LDA 0,1(7) save return in ac
147: LDA 7,-141(7) Relative jump to current func
148: LD 5,0(5) pop current frame
* swap
* if: jump to end belongs here
137: JEQ 0,12(7) if: jmp to else
149: LDA 7,0(7) jmp to end
* <- if
* swap
* -> Id
150: LD 0,-2(5) Load array param address into ac
* <- Id
151: ST 0,-11(5) adding arg to activation record
* -> Id
152: LD 0,-6(5) Load value into the ac
* <- Id
153: ST 0,-12(5) adding arg to activation record
* -> Op
* -> Id
154: LD 0,-4(5) Load value into the ac
* <- Id
155: ST 0,-13(5) op: push left
* -> Const
156: LDC 0,1(0) load const
* <- Const
157: LD 1,-13(5) op: load left
158: SUB 0,1,0 op -
* <- Op
159: ST 0,-13(5) adding arg to activation record
160: ST 5,-9(5) store current fp
161: LDA 5,-9(5) push new frame
162: LDA 0,1(7) save return in ac
163: LDA 7,-157(7) Relative jump to current func
164: LD 5,0(5) pop current frame
* swap
* -> Id
* -> Op
* -> Id
165: LD 0,-4(5) Load value into the ac
* <- Id
166: ST 0,-9(5) op: push left
* -> Const
167: LDC 0,1(0) load const
* <- Const
168: LD 1,-9(5) op: load left
169: SUB 0,1,0 op -
* <- Op
170: LD 1,-2(5) Load array param address into ac1
171: SUB 0,1,0 Add index to base array address
172: LD 0,0(0) Loading value of array index into ac
* <- Id
173: LD 7,-1(5) load return pointer into pc
174: LD 7,-1(5) load return pointer into pc
* partition
* quicksort
175: ST 0,-1(5) store the return pointer
* -> if
* -> Op
* -> Op
* -> Id
176: LD 0,-3(5) Load value into the ac
* <- Id
177: ST 0,-8(5) op: push left
* -> Const
178: LDC 0,2(0) load const
* <- Const
179: LD 1,-8(5) op: load left
180: ADD 0,1,0 op +
* <- Op
181: ST 0,-8(5) op: push left
* -> Id
182: LD 0,-4(5) Load value into the ac
* <- Id
183: LD 1,-8(5) op: load left
184: SUB 0,1,0 op <
185: JLT 0,2(7) br if true
186: LDC 0,0(0) false case
187: LDA 7,1(7) unconditional jmp
188: LDC 0,1(0) true case
* <- Op
* if: jump to else belongs here
* -> assign
* -> Id
190: LDA 0,-9(5) Load address into the ac
* <- Id
191: ST 0,-12(5) =: push left address
* -> Const
192: LDC 0,0(0) load const
* <- Const
193: LD 1,-12(5) op: load left
194: ST 0,0(1) assign: store value
* <- assign
* -> assign
* -> Id
195: LDA 0,-8(5) Load address into the ac
* <- Id
196: ST 0,-12(5) =: push left address
* partition
* -> Id
197: LD 0,-2(5) Load array param address into ac
* <- Id
198: ST 0,-15(5) adding arg to activation record
* -> Id
199: LD 0,-3(5) Load value into the ac
* <- Id
200: ST 0,-16(5) adding arg to activation record
* -> Id
201: LD 0,-4(5) Load value into the ac
* <- Id
202: ST 0,-17(5) adding arg to activation record
203: ST 5,-13(5) store current fp
204: LDA 5,-13(5) push new frame
205: LDA 0,1(7) save return in ac
206: LDA 7,-168(7) Relative jump to current func
207: LD 5,0(5) pop current frame
* partition
208: LD 1,-12(5) op: load left
209: ST 0,0(1) assign: store value
* <- assign
* -> assign
* -> Id
210: LDA 0,-10(5) Load address into the ac
* <- Id
211: ST 0,-12(5) =: push left address
* -> Id
212: LD 0,-3(5) Load value into the ac
* <- Id
213: LD 1,-12(5) op: load left
214: ST 0,0(1) assign: store value
* <- assign
* -> assign
* -> Id
215: LDA 0,-11(5) Load address into the ac
* <- Id
216: ST 0,-12(5) =: push left address
* -> Op
* -> Id
217: LD 0,-4(5) Load value into the ac
* <- Id
218: ST 0,-13(5) op: push left
* -> Const
219: LDC 0,1(0) load const
* <- Const
220: LD 1,-13(5) op: load left
221: SUB 0,1,0 op -
* <- Op
222: LD 1,-12(5) op: load left
223: ST 0,0(1) assign: store value
* <- assign
* -> while
* while: test, if == 0 jmp after body
* -> Op
* -> Id
224: LD 0,-9(5) Load value into the ac
* <- Id
225: ST 0,-12(5) op: push left
* -> Const
226: LDC 0,0(0) load const
* <- Const
227: LD 1,-12(5) op: load left
228: SUB 0,1,0 op ==
229: JEQ 0,2(7) br if true
230: LDC 0,0(0) false case
231: LDA 7,1(7) unconditional jmp
232: LDC 0,1(0) true case
* <- Op
* -> assign
* -> Id
234: LDA 0,-10(5) Load address into the ac
* <- Id
235: ST 0,-12(5) =: push left address
* -> Op
* -> Id
236: LD 0,-10(5) Load value into the ac
* <- Id
237: ST 0,-13(5) op: push left
* -> Const
238: LDC 0,1(0) load const
* <- Const
239: LD 1,-13(5) op: load left
240: ADD 0,1,0 op +
* <- Op
241: LD 1,-12(5) op: load left
242: ST 0,0(1) assign: store value
* <- assign
* -> while
* while: test, if == 0 jmp after body
* -> Op
* -> Id
* -> Id
243: LD 0,-10(5) Load value into the ac
* <- Id
244: LD 1,-2(5) Load array param address into ac1
245: SUB 0,1,0 Add index to base array address
246: LD 0,0(0) Loading value of array index into ac
* <- Id
247: ST 0,-12(5) op: push left
* -> Id
248: LD 0,-8(5) Load value into the ac
* <- Id
249: LD 1,-12(5) op: load left
250: SUB 0,1,0 op <
251: JLT 0,2(7) br if true
252: LDC 0,0(0) false case
253: LDA 7,1(7) unconditional jmp
254: LDC 0,1(0) true case
* <- Op
* -> assign
* -> Id
256: LDA 0,-10(5) Load address into the ac
* <- Id
257: ST 0,-12(5) =: push left address
* -> Op
* -> Id
258: LD 0,-10(5) Load value into the ac
* <- Id
259: ST 0,-13(5) op: push left
* -> Const
260: LDC 0,1(0) load const
* <- Const
261: LD 1,-13(5) op: load left
262: ADD 0,1,0 op +
* <- Op
263: LD 1,-12(5) op: load left
264: ST 0,0(1) assign: store value
* <- assign
265: LDA 7,-23(7) while: jmp back to test
255: JEQ 0,10(7) while: jmp to end
* <- while
* -> assign
* -> Id
266: LDA 0,-11(5) Load address into the ac
* <- Id
267: ST 0,-12(5) =: push left address
* -> Op
* -> Id
268: LD 0,-11(5) Load value into the ac
* <- Id
269: ST 0,-13(5) op: push left
* -> Const
270: LDC 0,1(0) load const
* <- Const
271: LD 1,-13(5) op: load left
272: SUB 0,1,0 op -
* <- Op
273: LD 1,-12(5) op: load left
274: ST 0,0(1) assign: store value
* <- assign
* -> while
* while: test, if == 0 jmp after body
* -> Op
* -> Id
* -> Id
275: LD 0,-11(5) Load value into the ac
* <- Id
276: LD 1,-2(5) Load array param address into ac1
277: SUB 0,1,0 Add index to base array address
278: LD 0,0(0) Loading value of array index into ac
* <- Id
279: ST 0,-12(5) op: push left
* -> Id
280: LD 0,-8(5) Load value into the ac
* <- Id
281: LD 1,-12(5) op: load left
282: SUB 0,1,0 op >
283: JGT 0,2(7) br if true
284: LDC 0,0(0) false case
285: LDA 7,1(7) unconditional jmp
286: LDC 0,1(0) true case
* <- Op
* -> assign
* -> Id
288: LDA 0,-11(5) Load address into the ac
* <- Id
289: ST 0,-12(5) =: push left address
* -> Op
* -> Id
290: LD 0,-11(5) Load value into the ac
* <- Id
291: ST 0,-13(5) op: push left
* -> Const
292: LDC 0,1(0) load const
* <- Const
293: LD 1,-13(5) op: load left
294: SUB 0,1,0 op -
* <- Op
295: LD 1,-12(5) op: load left
296: ST 0,0(1) assign: store value
* <- assign
297: LDA 7,-23(7) while: jmp back to test
287: JEQ 0,10(7) while: jmp to end
* <- while
* -> if
* -> Op
* -> Id
298: LD 0,-10(5) Load value into the ac
* <- Id
299: ST 0,-12(5) op: push left
* -> Id
300: LD 0,-11(5) Load value into the ac
* <- Id
301: LD 1,-12(5) op: load left
302: SUB 0,1,0 op <
303: JLT 0,2(7) br if true
304: LDC 0,0(0) false case
305: LDA 7,1(7) unconditional jmp
306: LDC 0,1(0) true case
* <- Op
* if: jump to else belongs here
* swap
* -> Id
308: LD 0,-2(5) Load array param address into ac
* <- Id
309: ST 0,-14(5) adding arg to activation record
* -> Id
310: LD 0,-10(5) Load value into the ac
* <- Id
311: ST 0,-15(5) adding arg to activation record
* -> Id
312: LD 0,-11(5) Load value into the ac
* <- Id
313: ST 0,-16(5) adding arg to activation record
314: ST 5,-12(5) store current fp
315: LDA 5,-12(5) push new frame
316: LDA 0,1(7) save return in ac
317: LDA 7,-311(7) Relative jump to current func
318: LD 5,0(5) pop current frame
* swap
* if: jump to end belongs here
307: JEQ 0,12(7) if: jmp to else
* -> assign
* -> Id
320: LDA 0,-9(5) Load address into the ac
* <- Id
321: ST 0,-12(5) =: push left address
* -> Const
322: LDC 0,1(0) load const
* <- Const
323: LD 1,-12(5) op: load left
324: ST 0,0(1) assign: store value
* <- assign
319: LDA 7,5(7) jmp to end
* <- if
325: LDA 7,-102(7) while: jmp back to test
233: JEQ 0,92(7) while: jmp to end
* <- while
* swap
* -> Id
326: LD 0,-2(5) Load array param address into ac
* <- Id
327: ST 0,-14(5) adding arg to activation record
* -> Id
328: LD 0,-10(5) Load value into the ac
* <- Id
329: ST 0,-15(5) adding arg to activation record
* -> Op
* -> Id
330: LD 0,-4(5) Load value into the ac
* <- Id
331: ST 0,-16(5) op: push left
* -> Const
332: LDC 0,1(0) load const
* <- Const
333: LD 1,-16(5) op: load left
334: SUB 0,1,0 op -
* <- Op
335: ST 0,-16(5) adding arg to activation record
336: ST 5,-12(5) store current fp
337: LDA 5,-12(5) push new frame
338: LDA 0,1(7) save return in ac
339: LDA 7,-333(7) Relative jump to current func
340: LD 5,0(5) pop current frame
* swap
* quicksort
* -> Id
341: LD 0,-2(5) Load array param address into ac
* <- Id
342: ST 0,-14(5) adding arg to activation record
* -> Id
343: LD 0,-3(5) Load value into the ac
* <- Id
344: ST 0,-15(5) adding arg to activation record
* -> Op
* -> Id
345: LD 0,-10(5) Load value into the ac
* <- Id
346: ST 0,-16(5) op: push left
* -> Const
347: LDC 0,1(0) load const
* <- Const
348: LD 1,-16(5) op: load left
349: SUB 0,1,0 op -
* <- Op
350: ST 0,-16(5) adding arg to activation record
351: ST 5,-12(5) store current fp
352: LDA 5,-12(5) push new frame
353: LDA 0,1(7) save return in ac
354: LDA 7,-180(7) Relative jump to current func
355: LD 5,0(5) pop current frame
* quicksort
* quicksort
* -> Id
356: LD 0,-2(5) Load array param address into ac
* <- Id
357: ST 0,-14(5) adding arg to activation record
* -> Op
* -> Id
358: LD 0,-10(5) Load value into the ac
* <- Id
359: ST 0,-15(5) op: push left
* -> Const
360: LDC 0,1(0) load const
* <- Const
361: LD 1,-15(5) op: load left
362: ADD 0,1,0 op +
* <- Op
363: ST 0,-15(5) adding arg to activation record
* -> Id
364: LD 0,-4(5) Load value into the ac
* <- Id
365: ST 0,-16(5) adding arg to activation record
366: ST 5,-12(5) store current fp
367: LDA 5,-12(5) push new frame
368: LDA 0,1(7) save return in ac
369: LDA 7,-195(7) Relative jump to current func
370: LD 5,0(5) pop current frame
* quicksort
* if: jump to end belongs here
189: JEQ 0,182(7) if: jmp to else
* -> assign
* -> Id
372: LDA 0,-8(5) Load address into the ac
* <- Id
373: ST 0,-9(5) =: push left address
* -> Op
* -> Op
* -> Id
374: LD 0,-3(5) Load value into the ac
* <- Id
375: ST 0,-10(5) op: push left
* -> Id
376: LD 0,-4(5) Load value into the ac
* <- Id
377: LD 1,-10(5) op: load left
378: ADD 0,1,0 op +
* <- Op
379: ST 0,-10(5) op: push left
* -> Const
380: LDC 0,2(0) load const
* <- Const
381: LD 1,-10(5) op: load left
382: DIV 0,1,0 op /
* <- Op
383: LD 1,-9(5) op: load left
384: ST 0,0(1) assign: store value
* <- assign
* -> if
* -> Op
* -> Id
* -> Id
385: LD 0,-3(5) Load value into the ac
* <- Id
386: LD 1,-2(5) Load array param address into ac1
387: SUB 0,1,0 Add index to base array address
388: LD 0,0(0) Loading value of array index into ac
* <- Id
389: ST 0,-9(5) op: push left
* -> Id
* -> Id
390: LD 0,-8(5) Load value into the ac
* <- Id
391: LD 1,-2(5) Load array param address into ac1
392: SUB 0,1,0 Add index to base array address
393: LD 0,0(0) Loading value of array index into ac
* <- Id
394: LD 1,-9(5) op: load left
395: SUB 0,1,0 op >
396: JGT 0,2(7) br if true
397: LDC 0,0(0) false case
398: LDA 7,1(7) unconditional jmp
399: LDC 0,1(0) true case
* <- Op
* if: jump to else belongs here
* swap
* -> Id
401: LD 0,-2(5) Load array param address into ac
* <- Id
402: ST 0,-11(5) adding arg to activation record
* -> Id
403: LD 0,-3(5) Load value into the ac
* <- Id
404: ST 0,-12(5) adding arg to activation record
* -> Id
405: LD 0,-8(5) Load value into the ac
* <- Id
406: ST 0,-13(5) adding arg to activation record
407: ST 5,-9(5) store current fp
408: LDA 5,-9(5) push new frame
409: LDA 0,1(7) save return in ac
410: LDA 7,-404(7) Relative jump to current func
411: LD 5,0(5) pop current frame
* swap
* if: jump to end belongs here
400: JEQ 0,12(7) if: jmp to else
412: LDA 7,0(7) jmp to end
* <- if
* -> if
* -> Op
* -> Id
* -> Id
413: LD 0,-3(5) Load value into the ac
* <- Id
414: LD 1,-2(5) Load array param address into ac1
415: SUB 0,1,0 Add index to base array address
416: LD 0,0(0) Loading value of array index into ac
* <- Id
417: ST 0,-9(5) op: push left
* -> Id
* -> Id
418: LD 0,-4(5) Load value into the ac
* <- Id
419: LD 1,-2(5) Load array param address into ac1
420: SUB 0,1,0 Add index to base array address
421: LD 0,0(0) Loading value of array index into ac
* <- Id
422: LD 1,-9(5) op: load left
423: SUB 0,1,0 op >
424: JGT 0,2(7) br if true
425: LDC 0,0(0) false case
426: LDA 7,1(7) unconditional jmp
427: LDC 0,1(0) true case
* <- Op
* if: jump to else belongs here
* swap
* -> Id
429: LD 0,-2(5) Load array param address into ac
* <- Id
430: ST 0,-11(5) adding arg to activation record
* -> Id
431: LD 0,-3(5) Load value into the ac
* <- Id
432: ST 0,-12(5) adding arg to activation record
* -> Id
433: LD 0,-4(5) Load value into the ac
* <- Id
434: ST 0,-13(5) adding arg to activation record
435: ST 5,-9(5) store current fp
436: LDA 5,-9(5) push new frame
437: LDA 0,1(7) save return in ac
438: LDA 7,-432(7) Relative jump to current func
439: LD 5,0(5) pop current frame
* swap
* if: jump to end belongs here
428: JEQ 0,12(7) if: jmp to else
440: LDA 7,0(7) jmp to end
* <- if
* -> if
* -> Op
* -> Id
* -> Id
441: LD 0,-8(5) Load value into the ac
* <- Id
442: LD 1,-2(5) Load array param address into ac1
443: SUB 0,1,0 Add index to base array address
444: LD 0,0(0) Loading value of array index into ac
* <- Id
445: ST 0,-9(5) op: push left
* -> Id
* -> Id
446: LD 0,-4(5) Load value into the ac
* <- Id
447: LD 1,-2(5) Load array param address into ac1
448: SUB 0,1,0 Add index to base array address
449: LD 0,0(0) Loading value of array index into ac
* <- Id
450: LD 1,-9(5) op: load left
451: SUB 0,1,0 op >
452: JGT 0,2(7) br if true
453: LDC 0,0(0) false case
454: LDA 7,1(7) unconditional jmp
455: LDC 0,1(0) true case
* <- Op
* if: jump to else belongs here
* swap
* -> Id
457: LD 0,-2(5) Load array param address into ac
* <- Id
458: ST 0,-11(5) adding arg to activation record
* -> Id
459: LD 0,-8(5) Load value into the ac
* <- Id
460: ST 0,-12(5) adding arg to activation record
* -> Id
461: LD 0,-4(5) Load value into the ac
* <- Id
462: ST 0,-13(5) adding arg to activation record
463: ST 5,-9(5) store current fp
464: LDA 5,-9(5) push new frame
465: LDA 0,1(7) save return in ac
466: LDA 7,-460(7) Relative jump to current func
467: LD 5,0(5) pop current frame
* swap
* if: jump to end belongs here
456: JEQ 0,12(7) if: jmp to else
468: LDA 7,0(7) jmp to end
* <- if
371: LDA 7,97(7) jmp to end
* <- if
469: LD 7,-1(5) load return pointer into pc
470: LD 7,-1(5) load return pointer into pc
* quicksort
* main
471: ST 0,-1(5) store the return pointer
* -> assign
* -> Id
472: LDA 0,-102(5) Load address into the ac
* <- Id
473: ST 0,-104(5) =: push left address
* -> Const
474: LDC 0,0(0) load const
* <- Const
475: LD 1,-104(5) op: load left
476: ST 0,0(1) assign: store value
* <- assign
* -> assign
* -> Id
477: LDA 0,-103(5) Load address into the ac
* <- Id
478: ST 0,-104(5) =: push left address
* -> Const
479: LDC 0,100(0) load const
* <- Const
480: LD 1,-104(5) op: load left
481: ST 0,0(1) assign: store value
* <- assign
* -> while
* while: test, if == 0 jmp after body
* -> Op
* -> Id
482: LD 0,-102(5) Load value into the ac
* <- Id
483: ST 0,-104(5) op: push left
* -> Const
484: LDC 0,100(0) load const
* <- Const
485: LD 1,-104(5) op: load left
486: SUB 0,1,0 op <
487: JLT 0,2(7) br if true
488: LDC 0,0(0) false case
489: LDA 7,1(7) unconditional jmp
490: LDC 0,1(0) true case
* <- Op
* -> assign
* -> Id
* -> Id
492: LD 0,-102(5) Load value into the ac
* <- Id
493: JGE 0,1(7) if array index < 0, halt
494: HALT 0,0,0 Negative array index
495: LDA 1,-2(5) Load array address into ac1
496: SUB 0,1,0 Add index to base array address
* <- Id
497: ST 0,-104(5) =: push left address
* -> Id
498: LD 0,-103(5) Load value into the ac
* <- Id
499: LD 1,-104(5) op: load left
500: ST 0,0(1) assign: store value
* <- assign
* -> assign
* -> Id
501: LDA 0,-102(5) Load address into the ac
* <- Id
502: ST 0,-104(5) =: push left address
* -> Op
* -> Id
503: LD 0,-102(5) Load value into the ac
* <- Id
504: ST 0,-105(5) op: push left
* -> Const
505: LDC 0,1(0) load const
* <- Const
506: LD 1,-105(5) op: load left
507: ADD 0,1,0 op +
* <- Op
508: LD 1,-104(5) op: load left
509: ST 0,0(1) assign: store value
* <- assign
* -> assign
* -> Id
510: LDA 0,-103(5) Load address into the ac
* <- Id
511: ST 0,-104(5) =: push left address
* -> Op
* -> Id
512: LD 0,-103(5) Load value into the ac
* <- Id
513: ST 0,-105(5) op: push left
* -> Const
514: LDC 0,1(0) load const
* <- Const
515: LD 1,-105(5) op: load left
516: SUB 0,1,0 op -
* <- Op
517: LD 1,-104(5) op: load left
518: ST 0,0(1) assign: store value
* <- assign
519: LDA 7,-38(7) while: jmp back to test
491: JEQ 0,28(7) while: jmp to end
* <- while
* quicksort
* -> Id
520: LDA 0,-2(5) Load array address into ac
* <- Id
521: ST 0,-106(5) adding arg to activation record
* -> Const
522: LDC 0,0(0) load const
* <- Const
523: ST 0,-107(5) adding arg to activation record
* -> Const
524: LDC 0,99(0) load const
* <- Const
525: ST 0,-108(5) adding arg to activation record
526: ST 5,-104(5) store current fp
527: LDA 5,-104(5) push new frame
528: LDA 0,1(7) save return in ac
529: LDA 7,-355(7) Relative jump to current func
530: LD 5,0(5) pop current frame
* quicksort
* -> assign
* -> Id
531: LDA 0,-102(5) Load address into the ac
* <- Id
532: ST 0,-104(5) =: push left address
* -> Const
533: LDC 0,0(0) load const
* <- Const
534: LD 1,-104(5) op: load left
535: ST 0,0(1) assign: store value
* <- assign
* -> while
* while: test, if == 0 jmp after body
* -> Op
* -> Id
536: LD 0,-102(5) Load value into the ac
* <- Id
537: ST 0,-104(5) op: push left
* -> Const
538: LDC 0,100(0) load const
* <- Const
539: LD 1,-104(5) op: load left
540: SUB 0,1,0 op <
541: JLT 0,2(7) br if true
542: LDC 0,0(0) false case
543: LDA 7,1(7) unconditional jmp
544: LDC 0,1(0) true case
* <- Op
* -> Id
* -> Id
546: LD 0,-102(5) Load value into the ac
* <- Id
547: LDA 1,-2(5) Load array address into ac1
548: SUB 0,1,0 Add index to base array address
549: LD 0,0(0) Loading value of array index into ac
* <- Id
550: OUT 0,0,0 output ac
* -> assign
* -> Id
551: LDA 0,-102(5) Load address into the ac
* <- Id
552: ST 0,-104(5) =: push left address
* -> Op
* -> Id
553: LD 0,-102(5) Load value into the ac
* <- Id
554: ST 0,-105(5) op: push left
* -> Const
555: LDC 0,1(0) load const
* <- Const
556: LD 1,-105(5) op: load left
557: ADD 0,1,0 op +
* <- Op
558: LD 1,-104(5) op: load left
559: ST 0,0(1) assign: store value
* <- assign
560: LDA 7,-25(7) while: jmp back to test
545: JEQ 0,15(7) while: jmp to end
* <- while
561: LD 7,-1(5) load return pointer into pc
* main
* Calling sequence for main
3: LDA 5,0(5) push frame main
4: LDA 0,1(7) save return in ac for main
5: LDA 7,465(7) Relative jump to main
* End of execution.
6: HALT 0,0,0 Correct Halt
|

|
|